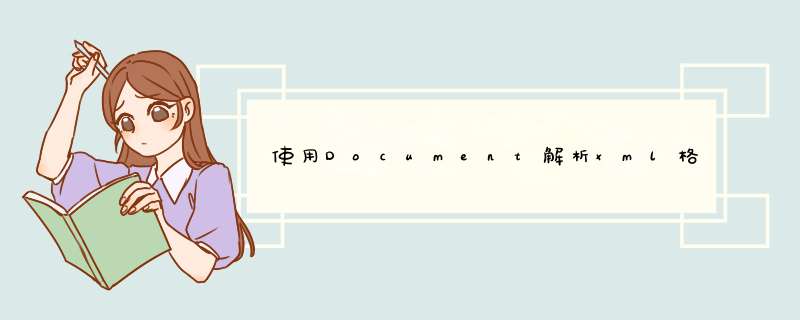
使用Document解析xml格式的文件(以P3C扫描结果为例)
一、xml文件格式(以P3C扫描结果为例)
二、示例代码
import org.w3c.dom.*;
import org.xml.sax.SAXException;
import javax.xml.parsers.documentBuilder;
import javax.xml.parsers.documentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class XmlTest2 {
public static void main(String[] args) {
String directory = "F:\p3c";
File file = new File(directory);
if (file.isDirectory()) {
File[] files = file.listFiles();
List
三、运行结果
{severity=BLOCKER, file=file://$PROJECT_DIR$/src/main/java/basicstudy/thread/ExecutorsTest.java, problem=线程池不允许使用Executors去创建,而是通过ThreadPoolExecutor的方式,这样的处理方式让写的同学更加明确线程池的运行规则,规避资源耗尽的风险。, functionName=basicstudy.thread.ExecutorsTest void newSingleThreadTest(), line=26, suggestion=手动创建线程池,效果会更好哦。 (line 26)}
{severity=BLOCKER, file=file://$PROJECT_DIR$/src/main/java/basicstudy/thread/ExecutorsTest.java, problem=线程池不允许使用Executors去创建,而是通过ThreadPoolExecutor的方式,这样的处理方式让写的同学更加明确线程池的运行规则,规避资源耗尽的风险。, functionName=basicstudy.thread.ExecutorsTest void newCachedThreadPoolTest(), line=75, suggestion=手动创建线程池,效果会更好哦。 (line 75)}
{severity=BLOCKER, file=file://$PROJECT_DIR$/src/main/java/basicstudy/thread/ExecutorsTest.java, problem=线程池不允许使用Executors去创建,而是通过ThreadPoolExecutor的方式,这样的处理方式让写的同学更加明确线程池的运行规则,规避资源耗尽的风险。, functionName=basicstudy.thread.ExecutorsTest void newFixedThreadPoolTest(), line=49, suggestion=手动创建线程池,效果会更好哦。 (line 49)}
四、说明
代码写的简单粗暴,没有经过优化,大家如有意见,欢迎评论,谢谢。
评论列表(0条)