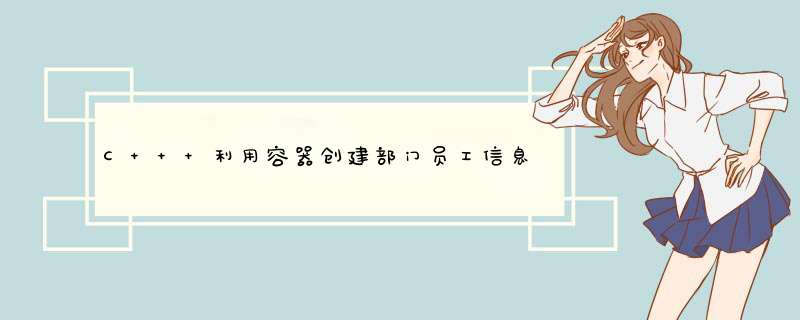
/*
公司招聘n个员工,(ABCDEFGH),员工进入公司后需要指派到各部门工作
员工信息有:姓名,年龄,工资;部门分为算法,运营,设计,产品
随机给员工分配部门和工资
通过multimap进行信息的插入,key(部门编号),value(员工)
分部门显示员工信息
*/
/*实现步骤
1.创建N名员工,放入到vector中
2.遍历vector容器,取出每个员工,进行随机分组
3.分组后将员工部门编号作为key,员工作为value,放入到multimap中
4.分部门显示员工信息
*/
#include
#include
评论列表(0条)