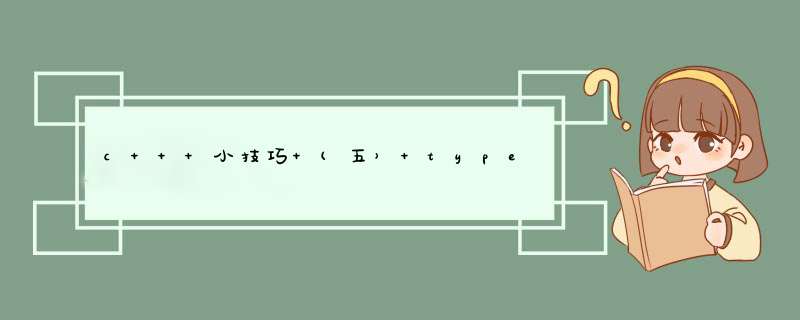
c++ 小技巧 (五) typedef
定义函数指针以及实例
1. typedef int* pint_t;//定义整形指针
2. //定义结构体,定义结构体指针
typedef struct {
double data;
}data_t, * pdata_t;
3. //定义函数指针,函数指针数组其实和函数指针差不多,看下面的例子
typedef void (*pfunction_t)();
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
评论列表(0条)