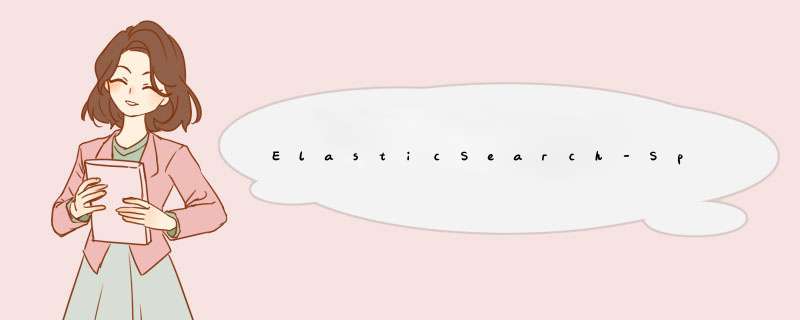
ElasticSearch-Spring Data ElasticSearch(RestHighLevelClient ) *** 作es(六)
本文使用的是springboot 2.2.x版本,elasticsearch使用的是6.8版本
官方文档参考:https://docs.spring.io/spring-data/elasticsearch/docs/4.2.7/reference/html/#new-features
对应版本
引入maven依赖
org.springframework.boot
spring-boot-starter-data-elasticsearch
springboot连接es的两种方式
方式一: 配置类
@Configuration
public class RestClientConfig {
@Bean
RestHighLevelClient client() {
ClientConfiguration clientConfiguration = ClientConfiguration.builder()
.connectedTo("填写es服务的ip+端口")
.build();
return RestClients.create(clientConfiguration).rest();
}
}
方式二:yml方式
spring:
elasticsearch:
rest:
uris: 106.52.98.231:19200
基于RestHighLevelClient 的入门例子
查询带高亮
更新
添加
删除
批量更新
使用ElasticSearchRepository进行基础查询
注意:spring data elasticsearch 在4.0版本后已经将@document注解的type属性弃用
使用ElasticSearchRepository自定义基本查询
语法形式
Keyword | Sample | Elasticsearch Query String |
---|
AndfindByNameAndPrice{"bool" : {"must" : [ {"field" : {"name" : "?"}}, {"field" : {"price" : "?"}} ]}}
OrfindByNameOrPrice{"bool" : {"should" : [ {"field" : {"name" : "?"}}, {"field" : {"price" : "?"}} ]}}
IsfindByName{"bool" : {"must" : {"field" : {"name" : "?"}}}}
NotfindByNameNot{"bool" : {"must_not" : {"field" : {"name" : "?"}}}}
BetweenfindByPriceBetween{"bool" : {"must" : {"range" : {"price" : {"from" : ?,"to" : ?,"include_lower" : true,"include_upper" : true}}}}}
LessThanEqualfindByPriceLessThan{"bool" : {"must" : {"range" : {"price" : {"from" : null,"to" : ?,"include_lower" : true,"include_upper" : true}}}}}
GreaterThanEqualfindByPriceGreaterThan{"bool" : {"must" : {"range" : {"price" : {"from" : ?,"to" : null,"include_lower" : true,"include_upper" : true}}}}}
BeforefindByPriceBefore{"bool" : {"must" : {"range" : {"price" : {"from" : null,"to" : ?,"include_lower" : true,"include_upper" : true}}}}}
AfterfindByPriceAfter{"bool" : {"must" : {"range" : {"price" : {"from" : ?,"to" : null,"include_lower" : true,"include_upper" : true}}}}}
LikefindByNameLike{"bool" : {"must" : {"field" : {"name" : {"query" : "?*","analyze_wildcard" : true}}}}}
StartingWithfindByNameStartingWith{"bool" : {"must" : {"field" : {"name" : {"query" : "?*","analyze_wildcard" : true}}}}}
EndingWithfindByNameEndingWith{"bool" : {"must" : {"field" : {"name" : {"query" : "*?","analyze_wildcard" : true}}}}}
Contains/ContainingfindByNameContaining{"bool" : {"must" : {"field" : {"name" : {"query" : "**?**","analyze_wildcard" : true}}}}}
InfindByNameIn
(Collectionnames){"bool" : {"must" : {"bool" : {"should" : [ {"field" : {"name" : "?"}}, {"field" : {"name" : "?"}} ]}}}}
NotInfindByNameNotIn
(Collectionnames){"bool" : {"must_not" : {"bool" : {"should" : {"field" : {"name" : "?"}}}}}}
NearfindByStoreNearNot Supported Yet !
TruefindByAvailableTrue{"bool" : {"must" : {"field" : {"available" : true}}}}
FalsefindByAvailableFalse{"bool" : {"must" : {"field" : {"available" : false}}}}
OrderByfindByAvailable
TrueOrderByNameDesc{"sort" : [{ "name" : {"order" : "desc"} }],"bool" : {"must" : {"field" : {"available" : true}}}}
如何使用?
public interface BookRepository extends ElasticsearchRepository {
//根据作者查询
List findByAuthor(String keyword);
//根据内容查询
List findByContent(String keyword);
//根据内容和名字查
List findByNameAndContent(String name,String content);
List findByNameAndContentAndPrice(String name,String content,Double price);
//根据内容或名称查询
List findByNameOrContent(String name,String content);
//范围查询
List findByPriceBetween(Double start,Double end);
//查询名字以xx开始的
List findByNameStartingWith(String name);
//查询某个字段值是否为false
List findByNameFalse();
//.......
}
使用RestHighLevelClient进行复杂高亮查询(ElasticsearchRepository不能满足高亮)
高亮
关于高亮字段输出的结果是这样子的
{detail=[detail], fragments[[好老师篮球篮球好篮球]], hobby=[hobby], fragments[[篮球]]}
评论列表(0条)